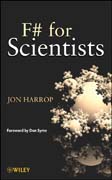
F# for Scientists explains and demonstrates the powerful features of this newprogramming language with a clear and concise style with useful examples throughout. It successfully strikes a balance between the functional aspects of F#and the object-oriented and task-based features that make F# so useful in practice. The book is well-suited for the computationally-oriented researcher, scientist, and developer. It is also appropriate as a textbook for advanced undergraduate and graduate students in various fields. INDICE: Foreword. Preface. Acknowledgments. List of Figures. List of Tables. Acronyms. 1. Introduction. 1.1 Programming guidelines. 1.2 A Brief History of F#. 1.3 Benefits of F#. 1.4 Introducing F#. 1.4.1 Language overview. 1.4.2 Pattern matching. 1.4.3 Equality. 1.4.4 Sequence expressions. 1.4.5 Exceptions. 1.5 Imperative programming. 1.6 Functional programming. 1.6.1 Immutability. 1.6.2 Recursion. 1.6.3 Higherorder functions. 1.6.4 Curried functions. 2. Program Structure. 2.1 Nesting. 2.2 Factoring. 2.2.1 Factoring out common subexpressions. 2.2.2 Factoring out higherorder functions. 2.3 Modules. 2.4 Objects. 2.4.1 Augmentations. 2.4.2 Classes. 2.5 Functional design patterns. 2.5.1 Combinators. 2.5.2 Maps and folds. 2.6 F# development. 2.6.1 Creating an F# project. 2.6.2 Building executables. 2.6.3 Debugging. 2.6.4 Interactive mode. 2.6.5 C# interoperability. 3. Data Structures. 3.1 Algorithmic Complexity. 3.1.1 Primitive operations. 3.1.2 Complexity. 3.2 Arrays. 3.2.1 Array literals. 3.2.2 Array indexing. 3.2.3 Array concatenation. 3.2.4 Aliasing. 3.2.5 Subarrays. 3.2.6 Creation. 3.2.7 Iteration. 3.2.8 Map. 3.2.9 Folds. 3.2.10 Sorting. 3.2.11 Pattern matching. 3.3 Lists. 3.3.1 Sorting. 3.3.2 Searching. 3.3.3 Filtering. 3.3.4 Maps and folds. 3.3.5 Pattern matching. 3.4 Sets. 3.4.1 Creation. 3.4.2 Insertion. 3.4.3 Cardinality. 3.4.4 Settheoretic operations. 3.4.5 Comparison. 3.5 Hash tables. 3.5.1 Creation. 3.5.2 Searching. 3.5.3 Insertion, replacement and removal. 3.5.4 Higherorder functions. 3.6 Maps. 3.6.1 Creation. 3.6.2 Searching. 3.6.3 Higherorder functions. 3.7 Choosing a data structure. 3.8 Seq. 3.9 Heterogeneous containers. 3.10 Trees. 3.10.1 Balanced trees. 3.10.2 Unbalanced trees. 3.10.3 Abstract Syntax Trees. 4. Numerical Analysis. 4.1 Number representation. 4.1.1 Machineprecision integers. 4.1.2 Machineprecision floatingpoint numbers. 4.2 Algebra. 4.3 Interpolation. 4.4 Quadratic solutions. 4.5 Meanand variance. 4.6 Other forms of arithmetic. 4.6.1 Arbitraryprecision integerarithmetic. 4.6.2 Arbitraryprecision rational arithmetic. 4.6.3 Adaptive precision. 5. Input and Output. 5.1 Printing. 5.1.1 Generating strings. 5.2 Generic printing. 5.3 Reading from and writing to files. 5.4 Serialization. 5.5 Lexing and Parsing. 5.5.1 Lexing. 5.5.2 Parsing. 6. Simple Examples. 6.1 Functional. 6.1.1 Nest. 6.1.2 Fixed point. 6.1.3 Within. 6.1.4 Memoize. 6.1.5 Binary search. 6.2 Numerical. 6.2.1 Heaviside step. 6.2.2 Kronecker ëfunction. 6.2.3 Gaussian. 6.2.4 Binomial coefficients. 6.2.5 Root finding. 6.2.6 Grad. 6.2.7 Function minimization. 6.2.8 Gamma function. 6.2.9 Discrete wavelet transform. 6.3 String related. 6.3.1 Transcribing DNA. 6.3.2 Word frequency. 6.4 List related. 6.4.1 count. 6.4.2 positions. 6.4.3 fold_to. 6.4.4 insert. 6.4.5 chop. 6.4.6 dice. 6.4.7 apply_at. 6.4.8 sub. 6.4.9 extract. 6.4.10 shuffle. 6.4.11 transpose. 6.4.12 combinations. 6.4.13 distribute. 6.4.14 permute. 6.4.15 Power set. 6.5 Array related. 6.5.1 rotate. 6.5.2 swap. 6.5.3 except. 6.5.4 shuffle. 6.6 Higherorder functions. 6.6.1 Tuple related. 6.6.2 Generalized products. 7. Visualization. 7.1 Windows Forms. 7.1.1 Forms. 7.1.2 Controls. 7.1.3 Events. 7.1.4 Bitmaps. 7.1.5 Example: Cellular automata. 7.1.6 Running an application. 7.2 Managed DirectX. 7.2.1 Handling DirectX devices. 7.2.2 Programmatic rendering. 7.2.3 Rendering an icosahedron. 7.2.4 Declarative rendering. 7.2.5 Spawning visualizations from the F# interactive mode. 7.3 Tesselating objects into triangles. 7.3.1 Spheres. 7.3.2 3D function plotting. 8. Optimization. 8.1 Timing. 8.1.1 Absolute time. 8.1.2 CPU time. 8.1.3 Looping. 8.1.4 Example timing. 8.2 Profiling. 8.2.1 8queens problem. 8.3 Algorithmic optimizations. 8.4 Lowerlevel optimizations. 8.4.1 Benchmarking data structures. 8.4.2 Compiler flags.8.4.3 Tailrecursion. 8.4.4 Avoiding allocation. 8.4.5 Terminating early. 8.4.6 Avoiding higherorder functions. 8.4.7 Use mutable. 8.4.8 Specialized functions. 8.4.9 Unboxing data structures. 8.4.10 Eliminate needless closures. 8.4.11Inlining. 8.4.12 Serializing. 9. Libraries. 9.1 Loading .NET libraries. 9.2 Charting and graphing. 9.3 Threads. 9.3.1 Thread safety. 9.3.2 Basic use. 9.3.3Locks. 9.3.4 The Thread Pool. 9.3.5 Asynchronous Delegates. 9.3.6 Background threads. 9.4 Random numbers. 9.5 Regular expressions. 9.6 Vectors and matrices. 9.7 Downloading from the Web. 9.8 Compression. 9.9 Handling XML. 9.9.1 Reading. 9.9.2 Writing. 9.9.3 Declarative representation. 9.10 Calling native libraries. 9.11 Fourier transform. 9.11.1 Nativecode bindings. 9.11.2 Interface in F#. 9.11.3 Pretty printing complex numbers. 9.11.4 Example use. 9.12 Metaprogramming. 9.12.1 Emitting IL code. 9.12.2 Compiling with LINQ. 10. Databases. 10.1 Protein Data Bank. 10.1.1 Interrogating the PDB. 10.1.2 Pretty printing XMLin F# interactive sessions. 10.1.3 Deconstructing XML using Active Patterns. 10.1.4 Visualization in a GUI. 10.2 Web Services. 10.2.1 US Temperature by ZipCode. 10.2.2 Interrogating the NCBI. 10.3 Relational Databases. 10.3.1 Connection to a database. 10.3.2 Executing SQL statements. 10.3.3 Evaluating SQL expressions. 10.3.4 Interrogating the database programmatically. 10.3.5 Filling the database from a data structure. 10.3.6 Visualizing the result. 10.3.7 Cleaning up. 11. Interoperability. 11.1 Excel interoperability. 11.1.1 Referencing the Excel interface. 11.1.2 Loading an existing spreadsheet. 11.1.3 Creating anew spreadsheet. 11.1.4 Referring to a worksheet. 11.1.5 Writing cell values into a worksheet. 11.1.6 Reading cell values from a worksheet. 11.2 MATLAB interoperability. 11.2.1 Creating a .NET interface from a COM interface. 11.2.2 Using the interface. 11.2.3 Remote execution of MATLAB commands. 11.2.4 Readingand writing MATLAB variables. 11.3 Mathematica interoperability. 11.3.1 Using.NETlink. 11.3.2 Example. 12. Complete Examples. 12.1 Fast Fourier Transform.12.1.1 Discrete Fourier Transform. 12.1.2 Radix 2 Fast Fourier Transform algorithm. 12.1.3 Bluesteins convolution algorithm. 12.1.4 Testing and Performance. 12.2 Semicircle Law. 12.2.1 Eigenvalue Computation. 12.2.2 Injecting resultsinto Excel. 12.2.3 Results. 12.3 Finding nthnearest neighbors. 12.3.1 Formulation. 12.3.2 Representing an atomic configuration. 12.3.3 Parser. 12.3.4 Lexer. 12.3.5 Main program. 12.3.6 Visualization. 12.4 Logistic map. 12.5 Realtime particle dynamics. Appendix A: Troubleshooting. A.1 Value restriction. A.2 Mutable array contents. A.3 Negative literals. A.4 Accidental capture. A.5 Local and nonlocal variable definitions. A.6 Merging lines. A.7 Applications that donot die. A.8 Beware of ‘it’. Glossary. Index.
- ISBN: 978-0-470-24211-7
- Editorial: John Wiley & Sons
- Encuadernacion: Cartoné
- Páginas: 376
- Fecha Publicación: 01/08/2008
- Nº Volúmenes: 1
- Idioma: Inglés